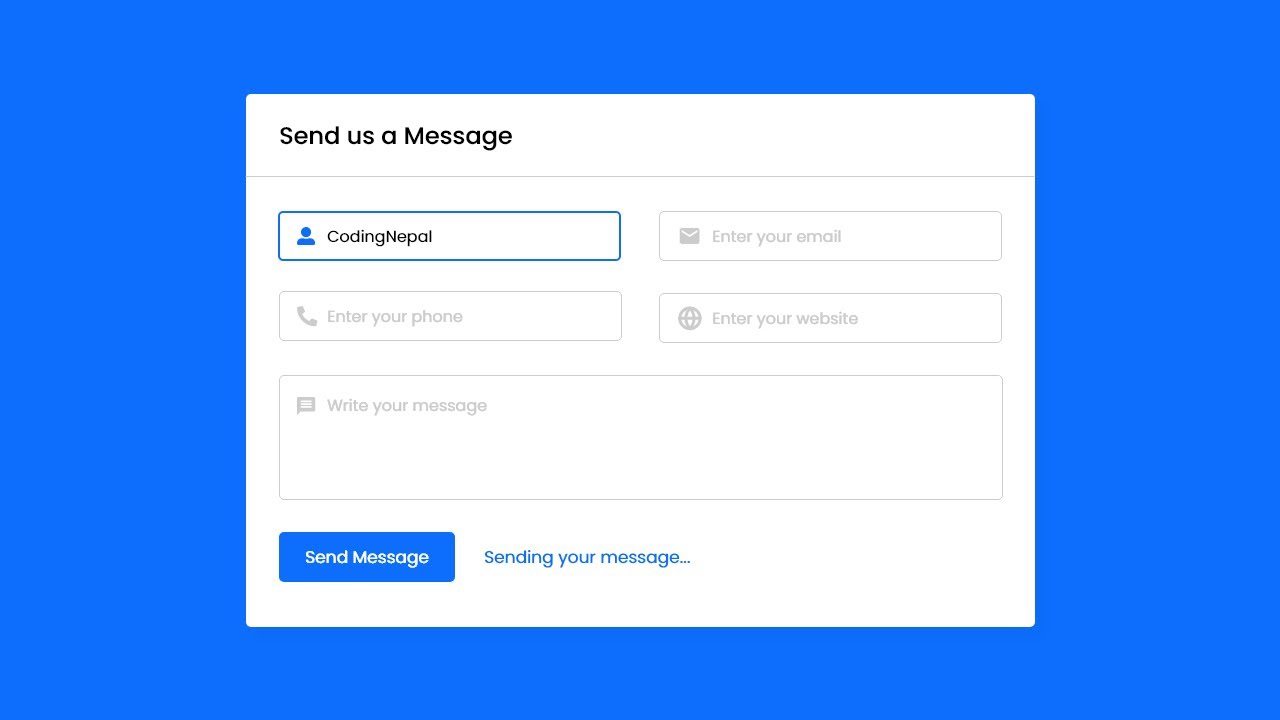
Creating an HTML and PHP contact form involves several steps:
-
- Create the HTML form:
- You can create an HTML form using basic HTML tags such as form, input, textarea, and button. Here’s an example:
<form method="post" action="contact.php">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="message">Message:</label>
<textarea id="message" name="message" required></textarea>
<button type="submit">Submit</button>
</form>
- Create the PHP script:
- The PHP script will handle the form data and send an email. You can create a PHP file named “contact.php” and add the following code:
<?php
if(isset($_POST['submit'])) {
// Get form data
$name = $_POST['name'];
$email = $_POST['email'];
$message = $_POST['message'];
// Set recipient email address
$to = "your-email@example.com";
// Set email subject
$subject = "New Contact Form Submission";
// Build the email content
$email_content = "Name: $name\n";
$email_content .= "Email: $email\n\n";
$email_content .= "Message:\n$message\n";
// Set email headers
$headers = "From: $name <$email>";
// Send the email
if(mail($to, $subject, $email_content, $headers)) {
// Redirect to thank-you page
header("Location: thank-you.html");
} else {
echo "Oops! Something went wrong. Please try again later.";
}
}
?>
- Test the form: Upload the HTML and PHP files to your web server and test the form by filling out the fields and submitting it. If everything works correctly, you should receive an email with the form data and be redirected to the thank-you page.
Note: Make sure to replace “your-email@example.com” with your own email address in the PHP script.
Leave a Reply