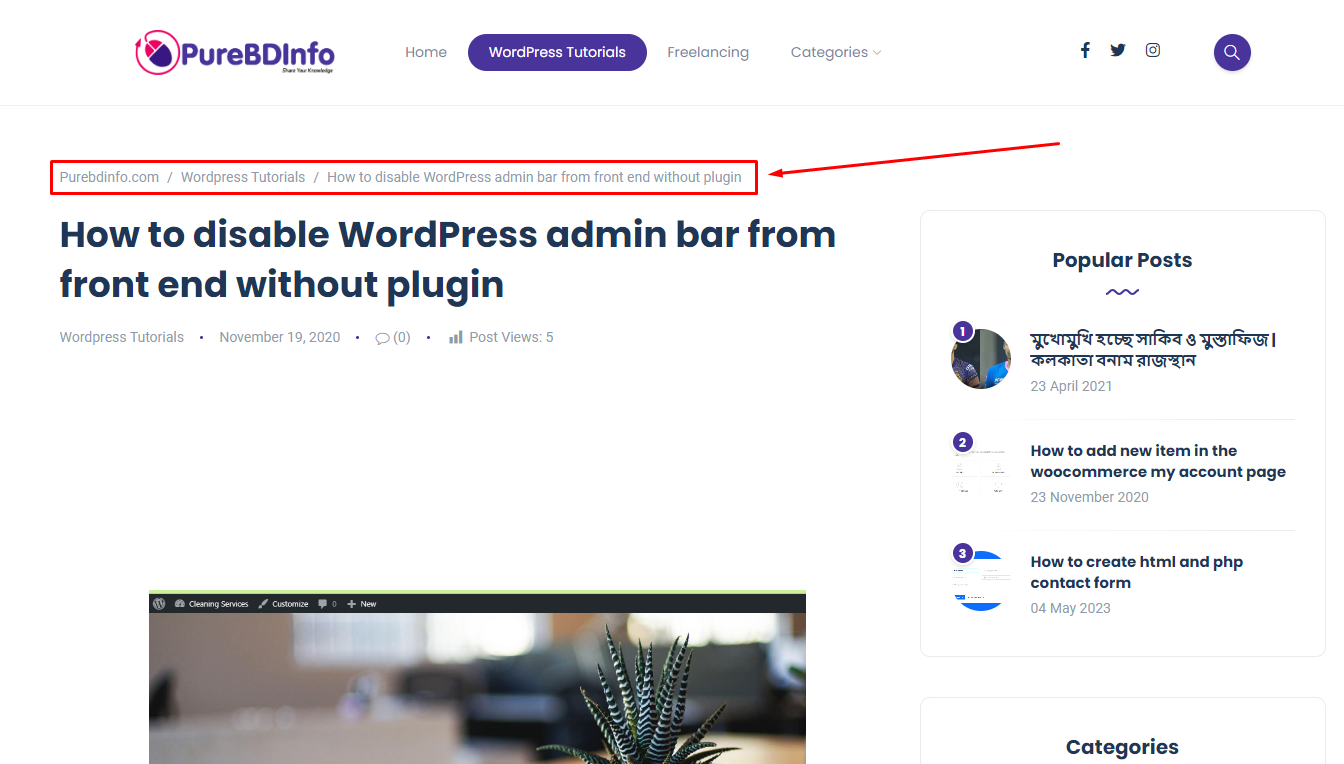
Here’s an example of creating a breadcrumb navigation for a WordPress website using PHP. You can limit the title characters in the breadcrumb navigation. Also, it is usable for all custom post types
<?php
function the_breadcrumb() {
// Set the separator character
$separator = "<div style='padding:0px 5px; '> › </div>";
// Set the character limit for titles
$title_limit = 30;
// Get the array of breadcrumb items
$breadcrumbs = array();
// Add the home link
$breadcrumbs[] = '<a href="' . get_home_url() . '">' . __('Home', 'text-domain') . '</a>';
// Check if it's a single post
if (is_singular('post')) {
$category = get_the_category();
if (!empty($category)) {
// Get the post category
$breadcrumbs[] = '<a href="' . get_category_link($category[0]->term_id) . '">' . $category[0]->name . '</a>';
}
// Add the current post title with character limit
$breadcrumbs[] = mb_strimwidth(get_the_title(), 0, $title_limit, '...');
}
// Check if it's a single page
elseif (is_singular('page')) {
// Get the ancestors of the current page
$ancestors = get_post_ancestors(get_the_ID());
// Reverse the array to start with the top-level parent
$ancestors = array_reverse($ancestors);
foreach ($ancestors as $ancestor) {
$breadcrumbs[] = '<a href="' . get_permalink($ancestor) . '">' . mb_strimwidth(get_the_title($ancestor), 0, $title_limit, '...') . '</a>';
}
// Add the current page title with character limit
$breadcrumbs[] = mb_strimwidth(get_the_title(), 0, $title_limit, '...');
}
// Check if it's a single post type
elseif (is_singular()) {
global $post;
$post_type = get_post_type_object($post->post_type);
if ($post_type->has_archive) {
$archive_link = get_post_type_archive_link($post->post_type);
$breadcrumbs[] = '<a href="' . $archive_link . '">' . $post_type->labels->name . '</a>';
}
// Add the current post type title with character limit
$breadcrumbs[] = mb_strimwidth(get_the_title(), 0, $title_limit, '...');
}
// Output the breadcrumb HTML
if (!empty($breadcrumbs)) {
echo '<div class="breadcrumb">' . implode(' ' . $separator . ' ', $breadcrumbs) . '</div>';
}
}
With this modification, the code will automatically detect the custom post type for single post type pages and generate the breadcrumb accordingly. It will check if the custom post type has an archive page, and if so, it will add a breadcrumb link to the archive page of the custom post type. Finally, it will display the current post type title.
Remember to call the the_breadcrumb()
function in your theme templates to display the breadcrumb navigation.
Leave a Reply